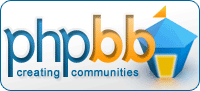 |
Castle Paradox
|
View previous topic :: View next topic |
Author |
Message |
RyuJinzo v(^_^)v
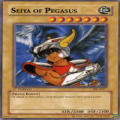
Joined: 15 Jun 2006 Posts: 55 Location: San Mateo, Rizal, Phillipines
|
Posted: Sat Jun 17, 2006 5:22 am Post subject: Endless moving (not walking) NPC plotscripts? |
|
|
Are there any plotscripts for endless moving NPCs? I mean, moving in their place but not walking just like in Dragon Quest/Warrior series?
Obviously speaking, I am one of avid fans of DQ/DW series. They're great!
Why not try playing them again? It's not bad to experience that old-school rpg feel...
Hail to the 6th warrior of Loto... _________________ St. SeiyaRyu
< Envoy Of Ur >
Last edited by RyuJinzo on Sat Jun 17, 2006 2:15 pm; edited 1 time in total |
|
Back to top |
|
 |
Onlyoneinall Bug finder
Joined: 16 Jul 2005 Posts: 746
|
Posted: Sat Jun 17, 2006 1:18 pm Post subject: |
|
|
Yeah, what you actually can do is place Step On NPCs around the NPC. He won't be able to move out of the space, but he'll keep moving. Unfortunately, he'll move really fast and look all over the place, depending on his settings. That's the only way I know out of plotscripting. _________________ http://www.castleparadox.com/gamelist-display.php?game=750 Bloodlust Demo 1.00
 |
|
Back to top |
|
 |
Iblis Ghost Cat

Joined: 26 May 2003 Posts: 1233 Location: Your brain
|
Posted: Sat Jun 17, 2006 2:10 pm Post subject: |
|
|
This is actually pretty easy to do in a plotscript. Here's what you do:
- Write a for loop that goes from 0 to 35. Make it set each NPC's frame to 0.
- Write another for loop that goes from 0 to 3. Make it set each Hero's frame to 0.
- Wait. Change the wait value depending on how fast or slow you want them to step (I use 12 ticks myself).
- Write two more for loops exactly like the previous ones, except they set the frames to 1 instead of 0.
- Wait again for the same amount you just did.
- Put all of this inside a while loop that never ends (give it a condition of true or 1).
- Set this script as the autorun script on every map where you want this effect (all of them, I'd assume).
Also, please refrain from sticking huge, irrelevant images in your posts. _________________ Locked
OHR Piano |
|
Back to top |
|
 |
RyuJinzo v(^_^)v
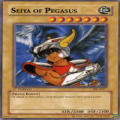
Joined: 15 Jun 2006 Posts: 55 Location: San Mateo, Rizal, Phillipines
|
Posted: Sat Jun 17, 2006 2:22 pm Post subject: Ouch! My head's hurts... |
|
|
I'm confused now...
Doing the plotscripts you have suggested me made me more confused. How are they made? I mean, would you please show me the script/s in HSPEAK format like we do when we use HSSed? _________________ St. SeiyaRyu
< Envoy Of Ur > |
|
Back to top |
|
 |
Iblis Ghost Cat

Joined: 26 May 2003 Posts: 1233 Location: Your brain
|
Posted: Sat Jun 17, 2006 3:49 pm Post subject: |
|
|
Rather than just give you the script I'm going to explain to you how the parts work. Getting a script from someone that you don't understand is pretty useless.
Quote: | Write a for loop that goes from 0 to 35. Make it set each NPC's frame to 0. |
This is a for loop:
Code: |
For (counter, 1, 10, 1) do
(
#Commands go here
)
|
"counter" is a variable. Variables can hold numeric values. To use it, you have to declare it earlier in your script. Do that like this:
1 is the starting value. At the beginning of the loop the value of counter is set to 1.
10 is the final value of the loop.
1 is the step value.
How it works is, counter is set to equal 1, then all the commands in the section labelled "Commands go here" are executed. Counter is then incremented by the step value (1), and is now 2. The commands execute again. Counter is incremented to 3. And so on, until it reaches 10. When counter is incremented to 10, the commands run again, and the loop ends. The values of 1, 10, and 1 can be changed to any numbers you want. You can also name the variable "counter" just about anything you want.
The variable "counter" can also be used in the loop. This is how you set each NPC's and hero's frame:
Code: |
For (counter, 0, 35, 1) do
(
Set NPC Frame (counter, 0)
)
|
How this works is, counter starts at 0. So the first time the loop runs, it sets NPC 0's frame to 0. Then it increments to 1, and it sets NPC 1's frame to 0. And so on, to NPC #35. You can do the same thing for heros by using "For (counter, 0, 3, 1)" and "Set Hero Frame" instead.
Quote: | Wait. Change the wait value depending on how fast or slow you want them to step (I use 12 ticks myself). |
This is the easy part. The wait command just makes a pause between doing things. So in this script:
Code: |
#Do these things
Wait (12)
#Do that stuff
|
It will "Do these things," then wait 12 ticks, then "Do that stuff." A "tick" is the unit of time that the OHR uses which, unfortunately, varies slightly depending on the speed of the computer. So I can't say "a tick is always equal to such and such number of milliseconds." All you can really do is guess how many ticks you're going to need for a certain script, then try it out, and increase or decrease the wait depending on whether it's running too fast or too slow.
Quote: | Put all of this inside a while loop that never ends (give it a condition of true or 1). |
A while loop is different from a for loop. A for loop repeats its commands a known number of times, whereas a while loops repeats its commands until a specified condition is no longer true. So a for loop repeats for a certain number of times, and a while loops repeats while a condition is true. Here's how it works:
Code: |
While (num == 5) do
(
#Put commands here
Wait (1)
)
|
The loop would repeat "Put commands here" until num ceased to equal 5, meaning that somewhere in "Put commands here" is something that can change the value of num.
The reason the "Wait" command is there is that while loops need to have a wait in them or they don't work. For loops do fine without waiting, while loops do not.
You'll probably notice the two equal signs in the condition. "num == 5" instead of "num = 5." This is because in Hamsterpeak, "=" by itself doesn't really mean anything. I'm not sure why exactly this is, but it is. When you want to check whether two values are equal, use ==.
For your purposes, the while loop doesn't ever need to end, since people will always be walking in place. So here's what you do:
Code: |
While (True) do
(
#Make them walk in place here
)
|
"True" is a constant. It always has a value of true, it can't be changed. So the loop will never end.
So, your script will look basically like this:
Code: |
Declare your variable
While (true) do
(
For loop for the NPCs, set frame to 0
For loop for the Heroes, set frame to 0
Wait
For loop for the NPCs, set frame to 1
For loop for the Heroes, set frame to 1
Wait
)
|
Quote: | Set this script as the autorun script on every map where you want this effect (all of them, I'd assume). |
This part isn't even plotscripting. Once you finish your script, compile it, and import it into custom, go into each map's "general map data" and set this as the autorun script. _________________ Locked
OHR Piano |
|
Back to top |
|
 |
Artimus Bena Admiral
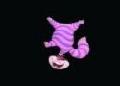
Joined: 17 Aug 2004 Posts: 637 Location: Dreamland.
|
Posted: Sat Jun 17, 2006 4:15 pm Post subject: |
|
|
I know this reply is kinda useless, but I wanted to say I appreciate that helpfulness hasn't been lost in CP.  _________________ SACRE BLEU!
|||Compositions!
|||Eldardeen Soundtrack!
|||Red Mercury! |
|
Back to top |
|
 |
RyuJinzo v(^_^)v
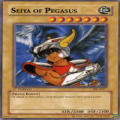
Joined: 15 Jun 2006 Posts: 55 Location: San Mateo, Rizal, Phillipines
|
Posted: Sat Jun 17, 2006 9:29 pm Post subject: |
|
|
Wow, that's a very big help I got from you, Sir Iblis. Since my thanks has nothing to do with your effort and time wasting just to help me figure out the plotscripting commands such as this. With your precise and detailed explanation of how-to-do's, I can now use this script in my game. How can I thank you? I think I'll have my turn helping you out. Again, thank you so much. For me this is a big appreciation to me. Ha hah!  _________________ St. SeiyaRyu
< Envoy Of Ur > |
|
Back to top |
|
 |
Mike Caron Technomancer

Joined: 26 Jul 2003 Posts: 889 Location: Why do you keep asking?
|
Posted: Sun Jun 18, 2006 11:51 am Post subject: |
|
|
I would like to add that the aforementioned script will only work when there is no other scripts running, and in addition, you should only set it on one map, or even better, set it to the new-game and load-game scripts. Otherwise, it'll trigger every time you switch maps, and will eventually overflow the script buffer.
Here's why _________________ I stand corrected. No rivers ran blood today. At least, none that were caused by us.
Final Fantasy Q
OHR Developer BLOG
Official OHRRPGCE Wiki and FAQ |
|
Back to top |
|
 |
TMC On the Verge of Insanity
Joined: 05 Apr 2003 Posts: 3240 Location: Matakana
|
Posted: Sun Jun 18, 2006 7:03 pm Post subject: |
|
|
Quote: | I would like to add that the aforementioned script will only work when there is no other scripts running, and in addition, you should only set it on one map, or even better, set it to the new-game and load-game scripts. Otherwise, it'll trigger every time you switch maps, and will eventually overflow the script buffer. |
I wish we there was some easy way around that. I tried my best to explain it at How do I write map autorun scripts? _________________ "It is so great it is insanely great." |
|
Back to top |
|
 |
Bob the Hamster OHRRPGCE Developer
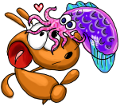
Joined: 22 Feb 2003 Posts: 2526 Location: Hamster Republic (Southern California Enclave)
|
Posted: Mon Jun 19, 2006 10:40 am Post subject: walking in place |
|
|
Hmmm.... you know, Dragon-Warrior-style Walking-in-place would really be very easy to build into the engine, just as another movement type. |
|
Back to top |
|
 |
satyrisci ~guo~

Joined: 28 Feb 2007 Posts: 73
|
Posted: Wed Mar 21, 2007 4:27 pm Post subject: |
|
|
Quote: | Hmmm.... you know, Dragon-Warrior-style Walking-in-place would really be very easy to build into the engine, just as another movement type. |
Please? It would be nice to use this feature to animate (for example) torches, so that you could place them wherever instead of having to make a separate animated maptile for each type of wall. |
|
Back to top |
|
 |
Bob the Hamster OHRRPGCE Developer
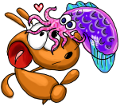
Joined: 22 Feb 2003 Posts: 2526 Location: Hamster Republic (Southern California Enclave)
|
Posted: Wed Mar 21, 2007 4:46 pm Post subject: |
|
|
satyrisci wrote: | Quote: | Hmmm.... you know, Dragon-Warrior-style Walking-in-place would really be very easy to build into the engine, just as another movement type. |
Please? It would be nice to use this feature to animate (for example) torches, so that you could place them wherever instead of having to make a separate animated maptile for each type of wall. |
I already added the feature months ago (just minutes after posting that comment) It will be in the next stable release. If you want to try it out now, you can download a 'nightly' |
|
Back to top |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|