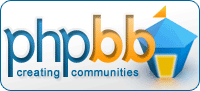 |
Castle Paradox
|
View previous topic :: View next topic |
Author |
Message |
msw188
Joined: 02 Jul 2003 Posts: 1041
|
Posted: Thu Nov 22, 2007 3:55 pm Post subject: Getting item ID's from the inventory into globals |
|
|
I've been constructing the basic framework for my coveted (by me) individualized item-menu system, and I need a pretty simple thing that I'm not sure exists. I'd like to take all of the items in the regular inventory and store their IDs one at a time into global variables. Right now the only way I can think of to do this is to cycle through all 255 items (I'm using item 0 for special purposes) and do something like this:
Code: |
variable(globalIndex)
globalIndex:=#start of global list reserved for this mess
for(i,1,255),do
begin
while(inventory(i)>>0),do
(writeglobal(globalIndex,i), deleteitem(i), increment(globalindex))
end
|
Does this seem workable? It doesn't seem terribly efficient, cycling though all of the items. I could run a check everytime to see if I've maxed out my global index (I know the total possible amount of items that will be in the inventory), but if one of them is item 255 that won't speed things up at all. Any other ideas?
Similarly, suppose I know I only have ONE item in the inventory, and I want to use it without the player seeing the menu. Looking at the plotdictionary I don't think this is currently possible. Or am I missing something completely? _________________ My first completed OHR game, Tales of the New World:
http://castleparadox.com/gamelist-display.php?game=161
This website link is for my funk/rock band, Euphonic Brew:
www.euphonicbrew.com |
|
Back to top |
|
 |
TMC On the Verge of Insanity
Joined: 05 Apr 2003 Posts: 3240 Location: Matakana
|
Posted: Thu Nov 22, 2007 6:00 pm Post subject: |
|
|
No, no. Do this instead:
Code: | script, save inventory, global index, begin
for (i, 0, 254) do, begin
write global (global index + i, inventory (i))
end
end |
(IRC, item 254 is the last item, and 0 the first ?)
Quote: | Similarly, suppose I know I only have ONE item in the inventory, and I want to use it without the player seeing the menu. Looking at the plotdictionary I don't think this is currently possible. Or am I missing something completely? |
We really need some item commands. _________________ "It is so great it is insanely great." |
|
Back to top |
|
 |
msw188
Joined: 02 Jul 2003 Posts: 1041
|
Posted: Thu Nov 22, 2007 6:25 pm Post subject: |
|
|
No, I'm afraid that that is not a good idea for me. You see, there will only ever be up to 8 (or 16 if I decide to use two menus) items in the inventory. I am trying to save a hero's item menu in a set of 8 (or 16) successive globals. Since I am going to have several heroes, your method would require several blocks of 255 globals, which I am not thrilled about using, much less defining. Plus, it makes it a pain to populate the item menues and attack menues on the fly, which I will be doing VERY often. Your idea seems more geared at saving the state of the inventory. That's not what I am trying to do. What I do need to do is discover what is in the inventory, and then put these things in specfic globals.
Another problem that is practically the same. Because battles can only reward items to the standard item menu, I'm planning on having an after-battle script always check the standard item menu to see if there is anything in it (normally it should always be empty). Do we have an easy way to do that, or am I just going to have to run the 254-item loop?
I thought that there were 256 items, 0-255, but I guess not. It doesn't matter, all of my numbers are just off by one. But item commands are sorely needed. A command to read item data would be incredible, as would a command to use item(itemID) or use item in slot (slot in inventory). Same thing for attacks. See bug 516.
PS: The command inventory() doesn't count items that are equipped, does it? I really hope not. _________________ My first completed OHR game, Tales of the New World:
http://castleparadox.com/gamelist-display.php?game=161
This website link is for my funk/rock band, Euphonic Brew:
www.euphonicbrew.com |
|
Back to top |
|
 |
TMC On the Verge of Insanity
Joined: 05 Apr 2003 Posts: 3240 Location: Matakana
|
Posted: Fri Nov 23, 2007 5:53 pm Post subject: |
|
|
Ah, right. Well assuming you mean 8 or 16 type of items, with variable amounts of each, which your code is attempting to handle, it would be great if you knew the maximum amount of globals it would take (otherwise having 99 of an item would be trouble):
Code: | variable(globalIndex, item count)
globalIndex:=#start of global list reserved for this mess
for (i,1,254) do, begin
item count := inventory (i)
if (item count) then (
writeglobal (globalIndex, i)
writeglobal (globalIndex + 1, item count)
deleteitem (i, item count)
globalIndex += 2
)
end
write global (globalIndex, 0)
|
The zero at the end is to mark the end of the items list. For 8 items, you need at most 17 globals, for 16 items, 33 globals.
There's no easy way to check battle rewards. Scanning all 254 item type shouldn't be all that slow. Equipped items are removed from the inventory. _________________ "It is so great it is insanely great." |
|
Back to top |
|
 |
msw188
Joined: 02 Jul 2003 Posts: 1041
|
Posted: Sun Nov 25, 2007 4:50 pm Post subject: |
|
|
Thanks for everything. I am NOT planning on allowing items to be groupable, which I always found counter-intuitive. Why should the hero only be able to carry eight types of items, but be able to carry 99 pieces of lead armor? So luckily I don't need to worry about the 99 limit, or anything related to that.
Is there a particular reason you're putting the zero at the end there? I am going to be using item ID zero to mean 'empty', which will be important for a couple things. Since I know beforehand how many heroes there are in the game, I can set aside the correct amount of globals and know where each list terminates. Did you have a set idea about the need for that final zero to mark the end that I am not thinking of?
Quote: | Equipped items are removed from the inventory |
Assuming that this means that the inventory command doesn't count them, this is beautiful. _________________ My first completed OHR game, Tales of the New World:
http://castleparadox.com/gamelist-display.php?game=161
This website link is for my funk/rock band, Euphonic Brew:
www.euphonicbrew.com |
|
Back to top |
|
 |
Moogle1 Scourge of the Seas Halloween 2006 Creativity Winner


Joined: 15 Jul 2004 Posts: 3377 Location: Seattle, WA
|
Posted: Sun Nov 25, 2007 9:17 pm Post subject: |
|
|
By the same token, though, why should the player be able to carry eight suits of armor when he can't pick up a ninth apple? _________________
|
|
Back to top |
|
 |
TMC On the Verge of Insanity
Joined: 05 Apr 2003 Posts: 3240 Location: Matakana
|
Posted: Sun Nov 25, 2007 9:20 pm Post subject: |
|
|
Well you could always reset all the globals to 0 before saving the inventory to them (which I guess you must be doing), but using a terminator is the normal approach. _________________ "It is so great it is insanely great." |
|
Back to top |
|
 |
msw188
Joined: 02 Jul 2003 Posts: 1041
|
Posted: Mon Nov 26, 2007 7:42 am Post subject: |
|
|
To Moogle:
Oh I agree that this system also seems strange in some ways, but a weighting system would be VERY difficult to implement along with the standard battle system. It would mean that each hero's menu list would have variable length, and I have no idea how I would manage to get that to work in battle. And so to me having no grouping at all is sort of the lesser of two evils. Mostly from a gameplay perspective - I am trying to remedy what turned out to be a somewhat glaring balance flaw in the later parts of my first game, (when it was supposed to be becoming harder!) where the player could buy incredible amounts of items to replenish anything they needed between battles.
To TMC:
Yes, any time I reassign items to the hero from the item inventory I go until I'm done, and then check to see if I filled him up. If not then I assign zeroes to all the rest of his slots. I actually do not do this very often; in fact, I think the only time it will happen is when the hero is selling things in a shop. As of right now, all other item loss/acquisition is handled one item at a time. _________________ My first completed OHR game, Tales of the New World:
http://castleparadox.com/gamelist-display.php?game=161
This website link is for my funk/rock band, Euphonic Brew:
www.euphonicbrew.com |
|
Back to top |
|
 |
|
|
You can post new topics in this forum You can reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|