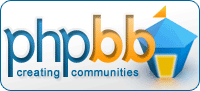 |
Castle Paradox
|
View previous topic :: View next topic |
Author |
Message |
Blacklight_Studios Guest
|
Posted: Fri Dec 03, 2010 11:11 am Post subject: I need help with my new game plotscripting... |
|
|
Okay, so i have the script written, for the most part. but i come across a problem:
the intended action of fading the screen out, then fading it back with a new text box isn't working correctly. It ends up being after an NPC triggered tutorial script placed at the hero's start point, which is fine, but when the script goes to fade the screen out, it shows the map screen before the fade. So how do I need to change in the script to fade the screen straight to black without flashing the map?
It would also help for you to know that the text boxes in question have backdrops on them to help enhance the plot. |
|
Back to top |
|
 |
JSH357
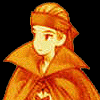
Joined: 02 Feb 2003 Posts: 1705
|
Posted: Fri Dec 03, 2010 11:23 am Post subject: |
|
|
(I'm assuming this is meant to happen when you start a new game?)
I would recommend not using the NPC activation method.
Go to your general game bitsets, then special plotscripts, and then set the script as your "new game script."
Another thing that might help is to use backdrop 0 (the black screen) or upload a black screen.
Then you can do this:
Code: | showbackdrop(0)
fadescreenout
suspendboxadvance
show text box (x)
show map
wait(20)
fadescreenin
resume box advance
wait for text box |
Backdrops display faster than fades, so if you want to start the game on a black screen, this is the best way. In fact, you could even kill the fade if you wanted and have:
Code: |
showbackdrop(0)
show text box (x)
wait for text box
show map
|
If you don't understand something there, please ask. Happy to help. |
|
Back to top |
|
 |
Blacklight_Studios Guest
|
Posted: Fri Dec 03, 2010 11:36 am Post subject: |
|
|
It seems that I should explain better, or I don't quite understand:
the NPC triggered script is the tutorial file. By whatever reason that script runs before the game intro script. This I'm okay with.
The game intro script is in fact loaded into the New Game option in the Special Plotscripts section of the General Game Data.
I guess it would be better to show the coding for my scripts and you tell me what's wrong with it.
Code: | plotscript, New Game, begin #this is the one set into New Game.
show text box (1)
fade screen in
wait for text box
show text box (2)
wait for text box
show text box (2)
fade screen out
show text box (3)
fade screen in
wait for text box
show text box (3)
fade screen out
show text box (4)
fade screen in
wait for text box
show text box (4)
fade screen out
resume player
fade screen in
end
#--------------------------------------------
plotscript, Game Intro, begin #this technically should be called Tutorial
suspend player
set hero direction (me, south)
show text box (5)
wait for text box
show text box (6)
wait for text box
show text box (7)
wait for text box
walk hero (me, north, 1)
wait for hero (me)
walk hero (me, south, 1)
wait for hero (me)
walk hero (me, east, 1)
wait for hero (me)
walk hero (me, west, 1)
wait for hero (me)
walk hero (me, south, 1)
wait for hero (me)
walk hero (me, north, 1)
wait for hero (me)
walk hero (me, west, 1)
wait for hero (me)
walk hero (me, east, 1)
wait for hero (me)
set hero direction (me, south)
show text box (8)
wait for text box
show text box (9)
wait for text box
show text box (10)
wait for text box
show text box (11)
fade screen out
end |
Now that I loot at it more, I think I see the problem, but I'd like your take on it. |
|
Back to top |
|
 |
Blacklight_Studios Guest
|
Posted: Fri Dec 03, 2010 11:41 am Post subject: |
|
|
it looks like I just need to add a showbackdrop command after the wait for text box that matches the text box... Opinion? |
|
Back to top |
|
 |
JSH357
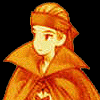
Joined: 02 Feb 2003 Posts: 1705
|
Posted: Fri Dec 03, 2010 12:00 pm Post subject: |
|
|
Correct me if I'm wrong, but does script #1 begin exactly where the second one ends?
If that's the case, it would be more efficient to take out the new game script entirely and just put the code from script #1 at the end of #2. If you don't want to do that you can just call the script by name in the first one, e.g.:
Code: | wait for text box
show text box (10)
wait for text box
show text box (11)
fade screen out
New Game
end
|
A side note: fading is pretty wonky sometimes. I would advise inserting a wait() if you want things to happen AFTER the fade is done. Sometimes the fade will be triggered at the same time as the next action, so adding a wait makes the wait happen at the same time instead.
For instance:
Code: |
fadescreenout
wait(4)
showtextbox(x)
fadescreenin
|
|
|
Back to top |
|
 |
Blacklight_Studios Guest
|
Posted: Fri Dec 03, 2010 12:05 pm Post subject: |
|
|
Yes, the script happens one after the other.
I didn't think the wait command could work like that. I'll try fixing it the way I think it'll work, then yours if my method doesnt work.
Thanks for the help! |
|
Back to top |
|
 |
TMC On the Verge of Insanity
Joined: 05 Apr 2003 Posts: 3240 Location: Matakana
|
Posted: Sat Dec 04, 2010 2:55 am Post subject: |
|
|
The screen fade commands cause endless confusion. Hopefully after reading this, you should understand what's going on.
The engine runs in a loop like this:
poll for new keyboard/mouse input
run scripts until a wait is encountered
process player input (move heroes), move NPCs, trigger textboxes, etc
redraw the screen
repeat
However, screen fades (fade screen in, fade screen out, update palette) are performed immediately when the command is reached, using the PREVIOUS screen state (which is currently being displayed). The engine pauses until they are finished (unfortunate). So if you write:
Code: | show textbox(1)
fade screen in
wait(1) |
Then first the engine changes the current textbox to #1, without redrawing the screen and then fades the palette in, using the previous frame where textbox #1 was not yet visible, and then redraws the screen with the textbox. So JSH's last code snippet is wrong. You probably instead want to write:
Code: | show textbox(1)
wait(1)
fade screen in |
One tick is enough.
Notice that when fading the screen out, the opposite happens. All of these have the same effect:
Code: | fade screen out
wait(1)
show textbox(1)
|
Code: | fade screen out
show textbox(1)
|
Code: | show textbox(1)
fade screen out
|
They fade out the screen before the textbox is displayed.
So JSH's corrected snippet is:
Code: | fade screen out
show textbox(x)
wait
fade screen in |
Also, there's a related problem with "teleport to map", "use door" (and also "fight formation", "use npc", "game over", "load menu", "load from slot", "reset game"): they cause an automatic wait(1) when run, meaning they cause the screen to be redrawn. When you use "teleport to map" and screen fades in proximity, things get pretty hard to understand.
Quote: | the NPC triggered script is the tutorial file. By whatever reason that script runs before the game intro script. |
Ouch, I hadn't realised this. That's unfortunate. We can't change that, or the way the fade commands work, without breaking a lot of games.
However aside from that problem, normally the new game script (and map autorun scripts) start running a 1-3 ticks before the automatic fade-in when entering a map (why oh why didn't James stick with one tick?). Therefore rather than JSH's complicated script involving backdrops, the following is all you need to show a textbox and then fade in the screen:
Code: | show textbox(1)
wait(3) |
I'm thinking about a General Preference Bitset that changes these commands to work in sane ways, but it may be a bad idea. It'll create quite a large incompatibility between scripts. _________________ "It is so great it is insanely great." |
|
Back to top |
|
 |
Blacklight_Studios Guest
|
Posted: Tue Dec 07, 2010 8:19 pm Post subject: |
|
|
huh, i'll try that. thanks for the tip.
update: I've taken the tutorial script out altogether, and put the text boxes to an NPC.
A new issue I've come across is with a different NPC, that disappears prematurely. i'm wanting it to be in place when Tag2=OFF, then disappear when the tag gets turned on, which ive set for when the hero get his first weapon item. for whatever reason, when i run the .rpg file, the NPCs are either missing, or when I "talk" to them, they disappear. They're more often missing. I do have the usable repeatedly bitset on. is there something I'm missing with the tags or bitsets? or is it the fact that i don't have a starting weapon item equipped to him, so the game defaults the weapon prematurely, if that makes sense? |
|
Back to top |
|
 |
Blacklight_Studios Guest
|
Posted: Tue Dec 07, 2010 9:00 pm Post subject: |
|
|
Sorry for the double post, guys.
I tried Mad Cacti's script suggestion, and the script now looks like this:
Code: | plotscript, New Game, begin
show text box (1)
fade screen in
wait (1)
wait for text box
fade screen out
wait (1)
show text box (2)
fade screen in
wait (1)
wait for text box
fade screen out
wait (1)
show text box (3)
fade screen in
wait (1)
wait for text box
fade screen out
wait (1)
show text box (4)
fade screen in
wait (1)
wait for text box
fade screen out
wait (1)
show map
fade screen in
wait (1)
end |
i'll try this in the game, now. Thanks, guys! |
|
Back to top |
|
 |
TMC On the Verge of Insanity
Joined: 05 Apr 2003 Posts: 3240 Location: Matakana
|
Posted: Tue Dec 07, 2010 10:59 pm Post subject: |
|
|
No no! You want to place waits BEFORE "fade screen in" and "fade screen out". (However since you already have a "wait for textbox" before each "fade screen out", you don't need an additional "wait (1)") _________________ "It is so great it is insanely great." |
|
Back to top |
|
 |
Blacklight_Studios Guest
|
Posted: Tue Dec 07, 2010 11:12 pm Post subject: |
|
|
So then, the code would look something like this?
Code: | show text box (1)
wait (1)
fade screen in
wait for text box
wait (1)
fade screen out
show text box (2)
wait (1)
# and so on and soforth... |
|
|
Back to top |
|
 |
Blacklight_Studios Guest
|
Posted: Tue Dec 07, 2010 11:18 pm Post subject: |
|
|
Urg... again with double-posting...
One more question with the script: would it be profitable for to add the "suspend player" command on this script? or would that create more problems than necessary? |
|
Back to top |
|
 |
Bob the Hamster OHRRPGCE Developer
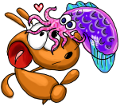
Joined: 22 Feb 2003 Posts: 2526 Location: Hamster Republic (Southern California Enclave)
|
Posted: Wed Dec 08, 2010 8:08 am Post subject: |
|
|
Blacklight_Studios wrote: | Urg... again with double-posting... ^^;
One more question with the script: would it be profitable for to add the "suspend player" command on this script? or would that create more problems than necessary? |
Yes, you usually want "suspend player" at the beginning and "resume player" at the end of every cutscene script. |
|
Back to top |
|
 |
Blacklight_Studios Guest
|
Posted: Wed Dec 08, 2010 9:53 am Post subject: |
|
|
Alright, I'll add that into the code...
it's still flashing the map whenever the textbox is terminated before the fade out... I'll try getting rid of the preceding wait commands and see what happens. |
|
Back to top |
|
 |
msw188
Joined: 02 Jul 2003 Posts: 1041
|
Posted: Wed Dec 08, 2010 10:19 am Post subject: |
|
|
Wait, are you saying you want the screen to fade out WITH THE OLD TEXT BOX STILL ON THE SCREEN, and then to fade back in with the new text box already in place? If that's the case, how will you know when to fade the screen (ie, how will you know when the player has finished reading the text box?)
If this is what you are trying to do, you will need to be familiar with the commands:
suspend box advance (and resume box advance)
advance text box
And if you want the player to trigger the fade without making the text box go away, you'll also need:
keypress commands like 'wait for key'
Let us know if this is the issue, and we can help you. _________________ My first completed OHR game, Tales of the New World:
http://castleparadox.com/gamelist-display.php?game=161
This website link is for my funk/rock band, Euphonic Brew:
www.euphonicbrew.com |
|
Back to top |
|
 |
|
|
You can post new topics in this forum You can reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|