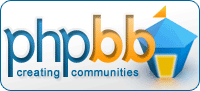 |
Castle Paradox
|
View previous topic :: View next topic |
Author |
Message |
Moogle1 Scourge of the Seas Halloween 2006 Creativity Winner


Joined: 15 Jul 2004 Posts: 3377 Location: Seattle, WA
|
Posted: Sat Dec 02, 2006 3:03 pm Post subject: Manual textbox script |
|
|
I'm writing a script to display text from textboxes. It uses string from textbox (from the nightly) to grab text from the box and displays it a letter at a time, oldschoolstyle. It also allows me to perform "special effects":
Code: | \n - newline
\w# - wait # ticks
\q - quit, end this textbox immediately
\p - pause, wait for usekey
\g### - go to textbox ###
\t### - if tag ### is true (followed by \q, \p, \w, \t, \T)
\T### - if tag ### is false (followed by \q, \p, \w, \t, \T) |
There are three issues with the script as it stands:
- String expansion (such as ${C0}) doesn't work. This is probably a bug in GAME.
- \p pauses the textbox like it should, but then it ends the textbox.
- The textbox's first line displays correctly. Subsequent lines chop off the first character.
For example, I'm using this textbox:
Code: | ${C0}\n "This is a worthy test of the
new \ntextbox feature. Don't you
\nagree?\p\g002 |
This displays the following:
Code: | {C0}
"This is a worthy test of the ew
textbox feature. Don't you n agree?" | (The goto fails.)
Here is the script. It should compile and run under the latest nightly as a standalone script.
Code: | define constant(32,ascii:space)
define constant(48,ascii:zero)
define constant(57,ascii:nine)
define constant(84,ascii:big_t)
define constant(92,ascii:backslash)
define constant(103,ascii:g)
define constant(110,ascii:n)
define constant(112,ascii:p)
define constant(112,ascii:q)
define constant(119,ascii:w)
define constant(116,ascii:t)
define constant(1,blankbox)
script, textbox, num (
variable(ctr)
variable(line) # line currently receiving text
variable(position) # char position in the textbox text
variable(textline) # line position in the textbox text
variable(char) # character buffer
variable(char2) # escaped character buffer (for numbers)
variable(done) # check if we are done
variable(number) # integer buffer for numbers
variable(do-next) # false if an if-tag has failed: cancels next command
line:=1
position:=1
textline:=0
done:=false
do-next:=true
for(ctr,1,9) do (clear string(ctr))
for(ctr,1,8) do (show string at(ctr,9,ctr*10--1))
string from textbox(9,num,textline,true)
show string at(9,0,100) #for testing purposes -- delete me
showtextbox(blankbox)
suspendboxadvance
while(done==false) do (
char:=ascii from string(9, position)
if (char==ascii:backslash) then ( #escape character!
position:=position+1
char:=ascii from string(9, position)
if (char==ascii:t) then ( #\t - if tag is ON
number:=0
for (ctr,0,2) do ( #3-digit number
position:=position+1
char2:=ascii from string(9, position)
if (char2>=ascii:zero && char2 <= ascii:nine) then
(number:=number*10+(char2--ascii:zero))
)
if (checktag(number)==false) then (do-next:=false)
)
if (char==ascii:big_t) then ( #\T - if tag is OFF
number:=0
for (ctr,0,2) do ( #3-digit number
position:=position+1
char2:=ascii from string(9, position)
if (char2>=ascii:zero && char2 <= ascii:nine) then
(number:=number*10+(char2--ascii:zero))
)
if (checktag(number)==true) then (do-next:=false)
)
if (char==ascii:n) then (line:=line+1) #\n - newline
if (char==ascii:p) then ( #\p - pause
if (do-next) then (waitforkey(usekey))
do-next:=true
)
if (char==ascii:w) then ( #\w - wait n ticks
position:=position+1
char2:=ascii from string(9, position)
if (do-next) then (wait(char2 -- ascii:zero))
do-next:=true
)
if (char==ascii:g) then ( #\g - goto textbox
number:=0
for (ctr,0,2) do ( #3-digit number
position:=position+1
char2:=ascii from string(9, position)
if (char2>=ascii:zero && char2 <= ascii:nine) then
(number:=number*10+(char2--ascii:zero))
)
if (do-next) then (
done:=true
textbox(number)
)
do-next:=true
)
if (char==ascii:q) then ( #\q - stop
if (do-next) then (done:=true)
do-next:=true
)
) else (append ascii(line,char)) #print the character in the buffer
position:=position+1
if (position>>string length(9)) then (
textline:=textline+1
position:=1
clear string(9)
if (textline<=7) then (stringfromtextbox(9,num,textline,true))
if (string length(9)==0) then (done:=true)
)
wait(1)
)
waitforkey(usekey)
for(ctr,0,7) do (
clear string(ctr+1)
hide string(ctr+1)
)
advancetextbox
resumeboxadvance
) |
Any thoughts? I'll keep hacking away at this, but I've made remarkably little progress in the last hour. (And, yes, before someone mentions it, I need to make \\ display a backslash. Script's not done yet.)
This script was a lot cleaner before I realized there was a 40-character limit on strings.  _________________
|
|
Back to top |
|
 |
Moogle1 Scourge of the Seas Halloween 2006 Creativity Winner


Joined: 15 Jul 2004 Posts: 3377 Location: Seattle, WA
|
Posted: Mon Dec 04, 2006 5:40 pm Post subject: |
|
|
I've debugged this, I think.
- String expansion (such as ${C0}) doesn't work. This is probably a bug in GAME.
This is still a bug, I think, but there's an easy workaround. (See '' . bug_title('273') . '')
- The textbox's first line displays correctly. Subsequent lines chop off the first character.
This is why ${C0} came out as {C0} (well, that and because of the other error). But this is also a bug -- ascii from string doesn't work like it should. (See '' . bug_title('274') . ''.)
- \p pauses the textbox like it should, but then it ends the textbox.
I accidentally gave ascii:q the value for ascii:p. Whoops.
Here is the updated script for anyone interested.
Code: | define constant(1, textboxwait)
define constant(32,ascii:space)
define constant(48,ascii:zero)
define constant(57,ascii:nine)
define constant(84,ascii:big_t)
define constant(92,ascii:backslash)
define constant(103,ascii:g)
define constant(110,ascii:n)
define constant(112,ascii:p)
define constant(113,ascii:q)
define constant(119,ascii:w)
define constant(116,ascii:t)
define constant(138,blankbox)
script, textbox, num (
variable(ctr)
variable(line) # line currently receiving text
variable(position) # char position in the textbox text
variable(textline) # line position in the textbox text
variable(char) # character being read
variable(char2) # characters being escaped
variable(done)
variable(number) # buffer for numbers
variable(do-next) # false if an if-tag has failed
line:=1
position:=0
textline:=0
done:=false
do-next:=true
for(ctr,1,9) do (clear string(ctr))
for(ctr,1,8) do (show string at(ctr,9,ctr*10--1))
string from textbox(9,num,textline)
expand string(9)
show string at(9,0,100)
showtextbox(blankbox)
suspendboxadvance
while(done==false) do (
char:=ascii from string(9, position)
if (char==ascii:backslash) then ( #escape character!
position:=position+1
char:=ascii from string(9, position)
if (char==ascii:t) then (
number:=0
for (ctr,0,2) do ( #3-digit number
position:=position+1
char2:=ascii from string(9, position)
if (char2>=ascii:zero && char2 <= ascii:nine) then
(number:=number*10+(char2--ascii:zero))
)
if (checktag(number)==false) then (do-next:=false)
)
if (char==ascii:big_t) then (
number:=0
for (ctr,0,2) do ( #3-digit number
position:=position+1
char2:=ascii from string(9, position)
if (char2>=ascii:zero && char2 <= ascii:nine) then
(number:=number*10+(char2--ascii:zero))
)
if (checktag(number)==true) then (do-next:=false)
)
if (char==ascii:n) then (line:=line+1)
if (char==ascii:p) then (
if (do-next) then (waitforkey(usekey))
do-next:=true
)
if (char==ascii:w) then (
position:=position+1
char2:=ascii from string(9, position)
if (do-next) then (wait(char2 -- ascii:zero))
do-next:=true
)
if (char==ascii:g) then (
number:=0
for (ctr,0,2) do ( #3-digit number
position:=position+1
char2:=ascii from string(9, position)
if (char2>=ascii:zero && char2 <= ascii:nine) then
(number:=number*10+(char2--ascii:zero))
)
if (do-next) then (
done:=true
textbox(number)
)
do-next:=true
)
if (char==ascii:q) then (
if (do-next) then (done:=true)
do-next:=true
)
) else (append ascii(line,char)) #print the character in the buffer
position:=position+1
if (position>>string length(9)) then (
textline:=textline+1
position:=0
clear string(9)
if (textline<=7) then (
string from textbox(9,num,textline)
expand string(9)
)
if (string length(9)==0) then (done:=true)
else (
wait(textboxwait)
append ascii(line,ascii:space)
)
)
wait(textboxwait)
)
for(ctr,0,7) do (
clear string(ctr+1)
hide string(ctr+1)
)
advancetextbox
resumeboxadvance
) |
_________________
|
|
Back to top |
|
 |
TMC On the Verge of Insanity
Joined: 05 Apr 2003 Posts: 3240 Location: Matakana
|
Posted: Fri Dec 08, 2006 2:00 am Post subject: |
|
|
(Whoops, slow reply)
Hey, I tried it out and that's pretty cool. I think what's really missing though is the ability to set the speed, and change it in the middle of a textbox.
...and what about being able to have more than 8 lines of text per textbox, and the text scrolling up when you go off the bottom? Would be neat.
BTW, the first string is string 0, not 1. 0-31 _________________ "It is so great it is insanely great." |
|
Back to top |
|
 |
Moogle1 Scourge of the Seas Halloween 2006 Creativity Winner


Joined: 15 Jul 2004 Posts: 3377 Location: Seattle, WA
|
Posted: Sat Dec 09, 2006 1:19 am Post subject: |
|
|
Thanks! The speed is a good suggestion. Unfortunately, there's not a lot between 0 and 1... 1 is already pretty slow. Maybe I should change it to characters per tick instead of ticks per character.
I like the scrolling suggestion. I'm not done with flags yet, of course; here are some others that I'm thinking about.
\s### and \S### - set and unset tag N, respectively
\f### - fight formation N
\m#### and \M#### - give and take N moneys
\$## - use shop N
\i### and \I### - give and take item N
\c - clear the text area, would be useful in combination with your suggestion
plus some scheme for a selection menu. Still working this one through in my head. I kind of also want the \p pause to display a "please push a button" cursor. I think that would be stylish. _________________
|
|
Back to top |
|
 |
Camdog
Joined: 08 Aug 2003 Posts: 606
|
Posted: Mon Dec 11, 2006 6:40 am Post subject: |
|
|
Wow, all those options give you a ton of power. Super rad. If you think of tags as an array of bits, you might even have created a turing-complete language withing OHR textboxes! It be a pain in the ass to code math operations, but still... meta-tastic! |
|
Back to top |
|
 |
Moogle1 Scourge of the Seas Halloween 2006 Creativity Winner


Joined: 15 Jul 2004 Posts: 3377 Location: Seattle, WA
|
Posted: Mon Dec 11, 2006 7:57 am Post subject: |
|
|
Wow! I didn't even think about that. If it's not already Turing-complete, I'm really tempted to make it now. _________________
|
|
Back to top |
|
 |
|
|
You can post new topics in this forum You can reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|