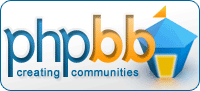 |
Castle Paradox
|
View previous topic :: View next topic |
Author |
Message |
mjkrzak

Joined: 24 Nov 2009 Posts: 43
|
Posted: Sun Oct 21, 2012 4:16 am Post subject: Setting global variables within a loop |
|
|
I'm looking for the correct syntax to set global variables via their ID number rather than their name.
Now, I know this doesn't work, but what it's supposed to do is systematically compare globals # 128-135, and make sure none of them are equal. If they are, randomly add -5 to 5 to the first compared value, set the test again flag, then do everything over again until no two values are the same.
So, what do I use in place of "global variable (count)" to get the job done? 'Cause I'm not seeing anything in the dictionary that fits what I need.
Quote: |
script, compare_rps_init, begin
varible (count1)
varible (count2)
varible (test)
test := 0
for(count1,128,134,1)
do(
for(count2,1,(135--count),1)
do(
if ((global variable (count)== global variable (count + count2))
then (
global variable (count) := global variable (count) + random (-5, 5)
test := 1
)
)
)
if (test == 1)
then(
compare_rps_init
)
end
|
|
|
Back to top |
|
 |
TMC On the Verge of Insanity
Joined: 05 Apr 2003 Posts: 3240 Location: Matakana
|
Posted: Sun Oct 21, 2012 4:58 am Post subject: |
|
|
The commands you want are 'read global' and 'write global'.
By the way, you could write your for loops more simply like so:
Code: | for (id1, 128, 134) do(
for (id2, id1 + 1, 134) do(
if (read global(id1) == read global(id2)) then (
...
)
)
) |
_________________ "It is so great it is insanely great." |
|
Back to top |
|
 |
mjkrzak

Joined: 24 Nov 2009 Posts: 43
|
Posted: Sun Oct 21, 2012 5:06 am Post subject: |
|
|
I tend to be happy enough that my code is usually functional. I don't practice it nearly often enough to make the evolution to elegant.
Thanks for the assist.
Oh, and before anyone says so, I already spotted my misspellings of variable. |
|
Back to top |
|
 |
sotrain515
Joined: 17 May 2010 Posts: 39 Location: Connecticut
|
Posted: Sun Oct 21, 2012 9:46 pm Post subject: Re: Setting global variables within a loop |
|
|
mjkrzak wrote: | I'm looking for the correct syntax to set global variables via their ID number rather than their name.
Now, I know this doesn't work, but what it's supposed to do is systematically compare globals # 128-135, and make sure none of them are equal. If they are, randomly add -5 to 5 to the first compared value, set the test again flag, then do everything over again until no two values are the same.
So, what do I use in place of "global variable (count)" to get the job done? 'Cause I'm not seeing anything in the dictionary that fits what I need.
Quote: |
script, compare_rps_init, begin
varible (count1)
varible (count2)
varible (test)
test := 0
for(count1,128,134,1)
do(
for(count2,1,(135--count),1)
do(
if ((global variable (count)== global variable (count + count2))
then (
global variable (count) := global variable (count) + random (-5, 5)
test := 1
)
)
)
if (test == 1)
then(
compare_rps_init
)
end
|
|
if I'm reading your code sample right, you are using recursion to do this... You should probably avoid that if possible, especially when there is an iterative solution available:
Code: |
variable(somediff, i, j)
somediff := true
while(somediff) do, begin
somediff := false
for(i, 128, 134) do, begin
for(j, i + 1, 134)do, begin
if(read global(i) == read global(j))then, begin
write global(i, read global(i) + random(-5, 5))
somediff := true
end
end
end
end
|
That way, it'll keep looping until until it doesn't find any differences.
Disclaimer: I didn't actually try running this code, but I do this kind of thing a lot in my code, so I think it'll work. |
|
Back to top |
|
 |
mswguest Guest
|
Posted: Tue Oct 23, 2012 5:15 pm Post subject: |
|
|
Doesn't this approach create a lot of wasted calculation? In fact, I wasn't even aware that Hspeak allowed a script to call itself (has this always been possible?).
It seems to me that this approach could very easily lend itself to potentially infinite loops. For one thing, every time you add "random(-5,5)", there's a chance you just added 0 so you haven't gained anything.
More importantly, with this approach, there's a chance that you keep changing things but never actually gain any ground in terms of unmatching variables. For example, imagine if all of the variables start out the same:
then our first step in the first pass changes the 2nd number:
Then our second step in the first pass changes the 3rd number (because it matches the 1st number):
then changes it again because we randomly made it match the 2nd number:
Hey! Wait a second, we just changed it back to match the 1st number! We can imagine that there is actually a chance that this process never manages to move the numbers away from each other in any meaningful fashion.
I would probably write the code to work on the global variables one at a time:
Code: | (pseudocode):
while(global2==global1),do
(global2:=global2+(-1)^random(1,2)*random(1,5))
while(global3==global1 ,or, global3==global2),do
(global3:=global3+same stuff)
while(global4==global1 ,or, global4==global2 ,or, global4==global3),do
etc |
Now there's also a chance that this process runs infinitely, but it seems to me to be a lot smaller of a chance, since we are fixing our globals one at a time, and the code 'knows when it is done messing with global3'. I'm not a computer scientist though; maybe one of the veterans of that field will be able to explain why my version actually makes no difference. |
|
Back to top |
|
 |
Bob the Hamster OHRRPGCE Developer
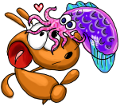
Joined: 22 Feb 2003 Posts: 2526 Location: Hamster Republic (Southern California Enclave)
|
Posted: Tue Oct 23, 2012 6:27 pm Post subject: |
|
|
mswguest wrote: | Doesn't this approach create a lot of wasted calculation? In fact, I wasn't even aware that Hspeak allowed a script to call itself (has this always been possible?). |
Yes, this has always been possible |
|
Back to top |
|
 |
TMC On the Verge of Insanity
Joined: 05 Apr 2003 Posts: 3240 Location: Matakana
|
Posted: Thu Oct 25, 2012 4:16 am Post subject: |
|
|
I would like to do an in-depth analysis, but probabilistic algorithm analysis is not my speciality, and this one is quite complex to analyse. Each element of the array is distributed differently and dependently: the first element can be changed up to 5 times through the function, while the last never is.
I would hope to estimate the running time by modelling the 5 array elements as random variables normally distributed around 0 (assuming the worst case where the initial values are all equal). Thanks to the Central Limit Theorem, this should be a decent approximation.
I did not complain about the use of recursion because intuitively the probability of a long running time is extremely small. The script interpreter allows up to 128 scripts running at once, so this is no problem. So msw's alternative is far too complex to justify.
But I figured I could do a quick simulation (starting from all-zero). Here's what I found:
Mean number of loops = 2.71 max over 5000 trials = 11
Increasing the array length to 20:
Mean number of loops = 8.20 max over 5000 trials = 43
Also, I looked at the distribution of values. Length 5 array:
Code: | Element 0 mean = -0.018 standard deviation = 6.59 max deviation over 5000 trials = 19
Element 1 mean = -0.022 standard deviation = 5.79 max deviation over 5000 trials = 15
Element 2 mean = -0.0272 standard deviation = 4.72 max deviation over 5000 trials = 10
Element 3 mean = -0.0364 standard deviation = 3.29 max deviation over 5000 trials = 5
Element 4 mean = 0.0 standard deviation = 0.00 max deviation over 5000 trials = 0
|
Increasing the size of the array to 12:
Code: | Element 0 mean = 0.1142 standard deviation = 10.80 max deviation over 5000 trials = 38
Element 1 mean = -0.2448 standard deviation = 10.30 max deviation over 5000 trials = 34
Element 2 mean = 0.1312 standard deviation = 9.74 max deviation over 5000 trials = 31
Element 3 mean = 0.0408 standard deviation = 9.26 max deviation over 5000 trials = 33
Element 4 mean = 0.029 standard deviation = 8.62 max deviation over 5000 trials = 28
Element 5 mean = 0.0838 standard deviation = 7.89 max deviation over 5000 trials = 27
Element 6 mean = 0.0194 standard deviation = 7.33 max deviation over 5000 trials = 22
Element 7 mean = 0.0684 standard deviation = 6.66 max deviation over 5000 trials = 20
Element 8 mean = 0.0892 standard deviation = 5.73 max deviation over 5000 trials = 15
Element 9 mean = -0.0234 standard deviation = 4.69 max deviation over 5000 trials = 10
Element 10 mean = -0.109 standard deviation = 3.34 max deviation over 5000 trials = 5
Element 11 mean = 0.0 standard deviation = 0.00 max deviation over 5000 trials = 0
|
Notice something interesting? The max deviations of the last variables goes up in steps of 5 because the last value never changes, the second last changes away from zero at most once, etc. Also, the standard deviation of the second last variable is of course sqrt((1+4+9+16+25)/5) = 3.32. Exercise for the reader: find closed forms for more of the standard deviations. _________________ "It is so great it is insanely great." |
|
Back to top |
|
 |
Bob the Hamster OHRRPGCE Developer
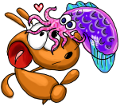
Joined: 22 Feb 2003 Posts: 2526 Location: Hamster Republic (Southern California Enclave)
|
Posted: Thu Oct 25, 2012 6:26 am Post subject: |
|
|
That is interesting, TMC.
Actually measuring speed is always better than just guessing at it :)
I am really curious how these numbers are actually being used in the game. What is their purpose? |
|
Back to top |
|
 |
Guest
|
Posted: Sun Oct 28, 2012 9:49 pm Post subject: |
|
|
This strikes me as far too much analysis for what I intend to use it for, but we do find our fun in the oddest places so...
The ultimate goal of this is to make a simplified battle system in a semi-plug in form. Like a multi-layered rock/paper/scissors dealie. I make it work, release it into the wild, then modify it again to my own giant golem castles slugfest minigame for my likely never to be finished RPG.
As it stands, the script is a simply a double check on the initial initiative scores. Each of the up to 8 targets gets a randomized value of 100-1000 + a speed score. (Don't want it to go negative, as I use -1 for targets not in play.) Once given, I run the checker to make sure no two values are the same. If they are, bump one and double check again. Repeat as needed, and use the numbers to determine who goes first-next-last.
As I see it, 8 randomized values in a field of 900 are unlikely to duplicate. If they do, there's a 10 in 11 chance that it'll be corrected first run and better odds in subsequent runs. While there's a slight chance for things to go off the rails, I see the odds as insignificant for the amount of times I intend to call the script. What, once a fight for maybe a half dozen times in a playthrough? |
|
Back to top |
|
 |
mswguest Guest
|
Posted: Mon Oct 29, 2012 8:04 am Post subject: |
|
|
Yeah, I noticed later that I misread your code. Now I notice it's a lot like what I was doing but backwards (you 'fix' numbers starting from the back in some sense, since your final number is never changed and your 2nd to last is 'fixed' mostly by changing the preceding numbers). I also imagined all of the numbers fairly close together, not in a field of 900! I don't know why; I guess because the 'bumping' was in a range of 10, I figured you were trying to keep the numbers fairly close to each other...? I guess this still confuses me a bit. If the initiatives are random from the start, why 'bump'? Why not just reassign altogether? |
|
Back to top |
|
 |
mjkrzak

Joined: 24 Nov 2009 Posts: 43
|
Posted: Mon Oct 29, 2012 8:59 pm Post subject: |
|
|
My first response would probably be: "That's what I thought to do first and there's no real reason why I couldn't reassign."
The rationalizing answer would be: "They came up close in the first randomization, so they should be next to each other in sequence rather than potentially throwing off the rest of the shuffle."
If pressed, the fallback position from the rationalizing answer would be a grudging first response.
In the end the numbers don't mean much, as they all get translated into a simple 0-8 (0 for unused) order. |
|
Back to top |
|
 |
|
|
You can post new topics in this forum You can reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|